Posts Tagged ‘GUI’
Android: hexadecimal color input using an EditText
Posted by Dimitri | Filed under Featured, Programming
This Android programming tutorial shows how to create a simple application that takes the value of a hexadecimal color at an EditText field and displays it as an ImageView. In order to do so, this post goes into detail on how to correctly parse the EditText String as 32 bit hexadecimal Integer. Additionally, it explains the part of the code that constrains the characters between A to F and 0 to 9 at the EditText. Finally, it tries to solve some of the EditText problems when applying these constrains.
The application in this post has been tested on Android 2.1, 2.3 and 4.0.
So, here’s a screenshot of the example project application in action:
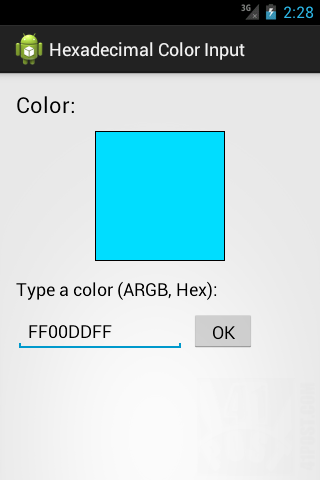
A screenshot of the example project. It’s available for download at the end of the post.
Unity: list files in a directory
Posted by Dimitri | Filed under Featured, Programming
This Unity3D programming post explains how to create a C# script that lists the files in a directory. Additionally, for the sake of completeness, this post also shows a rudimentary method that allows to select one of the files from the list and how to deal with some of the potential IO errors.
Here’s a screenshot of the code in action:
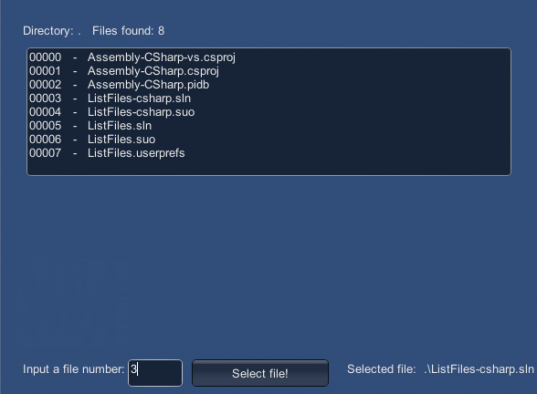
Unity: capturing audio from multiple microphones
Posted by Dimitri | Filed under Featured, Programming
As stated in the title, this Unity scripting tutorial explains how to select and record and playback audio from a list of connected microphones. It’s somewhat of a follow up of the previous post Unity: Capturing audio from a microphone. So, for those who are after a step by step explanation of how to capture audio from a single microphone in Unity, please read the previous post. The code featured in this tutorial has been developed and tested using Unity 3.5.4f1 at the editor and a as a standalone Windows application. A sample project with all the code discussed in this tutorial is available for download at the end of this tutorial.
Just as a reminder, to capture audio from a microphone in Unity, all that is necessary is to call the static Start() method from the Microphone class. It returns an AudioClip that can be played back using an AudioSource. However, this time, it’s now important to know how many microphones are connected to the computer and the recording capabilities of each microphone. This is going to be done by calling the static Microphone.GetDeviceCaps() method for each microphone and save the obtained information at two the integer arrays.
Unity: Capturing audio from a microphone
Posted by Dimitri | Filed under Programming
This Unity programming tutorial explains how to create a Unity script to capture the audio from a microphone. It also shows how to playback the captured audio and the necessary steps to check if there is a microphone present and its recording capabilities. The code featured in this post has been developed and tested using Unity 3.5.4f1 at the editor and a as a standalone Windows application. A sample project with all the code discussed in this tutorial is available for download at the end of the post.
To capture the audio input from a microphone in Unity, one can simply call the static Start() method from the Microphone class to start recording. This method returns an AudioClip that can be played back using an AudioSource. And that’s exactly what the script explained in this post will do. However, to avoid exceptions from being thrown, there is a simple verification to detect if there’s a microphone present prior to calling this method, and also, the mic audio capture capabilities are checked. Luckily, the same Microphone class also offers public members and methods that aid in accomplishing the two aforementioned tasks.
Unity: displaying the video input from multiple webcams
Posted by Dimitri | Filed under Programming
This post explains how to capture the images from a web camera connected to the computer and use them as a texture in Unity. However, this tutorial focuses on switching between the video inputs from different webcams attached to desktop computers (PC and Mac). However it should work on Android with some minor modifications. The code featured in this article has been developed and tested using a free license of Unity 3.5.3f3. As usual an example project with all the code featured in this article is available for download at the end of the post.
Before explaining how to choose from a list of multiple connected webcams to render from, let’s see how to preview the captured images from a single camera. Doing that in Unity is very simple and it can be achieved with just a few lines of code. Take a look at this C# script: (more…)