Android: Changing the animation between Activities
Posted by Dimitri | Mar 9th, 2011 | Filed under Programming
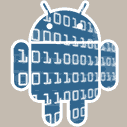
This post features how to change Android’s default animation when switching between Activities. Before reading the rest, please know that the code that changes the standard animation be found at the API Demo that comes with the Android SDK. But since there’s a lack of proper documentation regarding this subject and it’s difficult to find a place explaining it, here is a post that helps in aiding these two problems.
So, the code to change the animation between two Activities is very simple: just call the overridePendingTransition() from the current Activity, after starting a new Intent. This method is available from Android version 2.0 (API level 5), and it takes two parameters, that are used to define the enter and exit animations of your current Activity. Here’s an example:
//Calls a new Activity startActivity(new Intent(this, NewActivity.class)); //Set the transition -> method available from Android 2.0 and beyond overridePendingTransition(R.anim.push_left_in,R.anim.push_up_out);
These two parameters are resource IDs for animations defined with XML files (one for each animation). These files have to be placed inside the app’s res/anim folder. Examples of these files can be found at the Android API demo, inside the anim folder. Let’s take a look at one of these files (push_left_in.xml):
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="100%p" android:toXDelta="0" android:duration="300"/> <alpha android:fromAlpha="0.0" android:toAlpha="1.0" android:duration="300" /> </set>
As you can see on line 4, the screen is being translated from 100% of its parent width to 0 (meaning it is going from right to left). Line 5 makes adds a fade effect to the animation. It’s really simple to define a transition animation, it’s only a matter of calling the correct attribute and assigning a value to it. For a list of supported attributes for XML defined animations, click here. The 100%p value indicates that we want this attribute to be applied 100% relative to the parent. Other values could be 100% (without the “p“), which means relative to self or just a numeric value without suffixes making Android understand that we want this value to be interpreted as absolute.
One thing to watch out for is that, this code:
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="100%p" android:fromYDelta="100%p" android:toXDelta="0" android:toXDelta="0" android:duration="300"/> </set>
Is different from this one:
<?xml version="1.0" encoding="utf-8"?> <set xmlns:android="http://schemas.android.com/apk/res/android"> <translate android:fromXDelta="100%p" android:toXDelta="0" android:duration="150"/> <translate android:fromYDelta="100%p" android:toYDelta="0" android:duration="150"/> </set>
The first line of code makes slides the View diagonally. The second one slides the View to the left and then up. So, one must have in mind that all relative values are calculated based on the current position of the View on the screen.
Comments are always appreciated.
Thanks,
IT’s work in all sdk version ….
Great tutorial….keep it up….
Really? It’s a good thing to know. Although the overridePendingTransition() was made available from Android 2.0 and beyond. Is there a way to use it in 1.6?
Thanks!
Thanks, this post is very helpful.
Awesome tutorial.. Thanks for this tutorial…
Thanks its very helpful.
Thanks for this animation description. I agree the documentation of this at developers.android.com is really lacking.
The enter animation is for the incoming activity and exit animation is for the outgoing activity. Not the current activity.
Great article! Thanks :)
Awesome tutorial, thanks. I was thinking i could not do a transitional animation when using a canvas, but just noticed it has nothing to do with it =)
Awesome post man! Many ppl say they animate activity but only animates the layouts; U are Cool :)
Thanks,
It is much better than the Google tutorial, btw. that works only from the API 13
Cheers
Thanks for this post ..
Great Job:)-
which of this code will cum under push_up_out????
thanks for the great tutorial!
Indeed, awesome tutorials. Animation is annoying though.
How can I take a picture on the screen will move