Unity3D: JavaScript vs. C# – Part 3
Posted by Dimitri | Dec 4th, 2010 | Filed under Programming
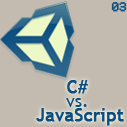
This is the third part of a series that show some of the differences between JavaScript and C# when writing scripts for Unity3D game engine. I suggest that you read the first and second post of the series to better understand what is going on here.
In this third part, I will point out some differences between JavaScript and C# by writing a script that makes a GameObject move forward. So, let’s start with the programming language that will take the smallest number of lines to make a GameObject move, JavaScript:
public var goTransform:Transform; private var vel:int = 2;//how fast the game object is being moved function Awake() { //get this GameObject's Transform goTransform = this.GetComponent(Transform); } // Update is called once per frame function Update() { //moves the containing GameObject forward goTransform.position.z = goTransform.position.z + vel; }
This script moves the attached game object forward. Note that it is possible to access and increment the goTransform’s position z property, every update cycle and that causes the GameObject to move forward. Let’s see how the same code is written using C#:
using UnityEngine; using System.Collections; public class PawnMover : MonoBehaviour { public Transform goTransform; private int vel = 2;//how fast the game object is being moved void Awake() { //get this GameObject's Transform goTransform = this.GetComponent<Transform>(); } // Update is called once per frame void Update() { //returns a CS1612 error goTransform.position.z=goTransform.position.z + vel;//<=returns a CS1612 error //this is the right way to do it when using C# goTransform.Translate(Vector3.forward * vel);//moves the containing GameObject forward } }
Here, C# can’t access the goTransform’s position z property, meaning that it can’t be incremented, like the JavaScript version of the above code. Trying to do it generates a CS1612 error, which means that we are trying to change a value directly and not a reference to that value. To avoid this error when writing scripts with C#, we have to use methods to move GameObjects, such as Translate(), Rotate(), RotateAround(), etc. All these methods are public members of the Transform class.
That’s it for this post. I hope I have shed some light in the main differences between these two programming languages, when writing scripts for Unity3D game engine. Don’t forget to check 41 Post for the fourth part of this series, where I will show some differences between JavaScript and C# when pausing code execution (a.k.a. yielding), which is available here: Part 4 – Yielding.
Isn’t the Transform type missing on the 12th line of C# version?
I mean:
goTransform = this.GetComponent();
instead of:
goTransform = this.GetComponent();
Anyway, very interesting post!
Yes, it is! Thanks! Just fixed that.
Fail… I meant
goTransform = this.GetComponent();
Oh ok inferior/superior brackets are stripped by the posting algorithm… XD
coding of drag and drop the object using mouse control