Unity3D: JavaScript vs. C# – Part 1
Posted by Dimitri | Nov 5th, 2010 | Filed under Featured, Programming
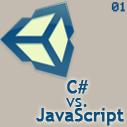
This is the first post of a series that will discuss some differences between JavaScript and C# when programming for Unity3D game engine. A project with all the scripts used in these posts will be available for download in the last post of the series. So let’s start from the start and see some of the main differences between the two languages.
The first and most easily distinguishable is the notation used to declare variables and methods. When programming in JavaScript, it isn’t necessary to declare the variable or method type whether in C#, it’s a must. That is because JavaScript is a weakly typed language, meaning that the interpreter chooses the best type to use at compilation-time. C# is a totally different because it is strongly typed, so, the type has to be declared before a variable or method. Let’s see some examples to better grasp this concept. The following script is a JavaScript example:
private var cubeTransform;
And in the C#, the same code would be:
private Transform cubeTransform;
This is also valid for methods. Even if a C# method returns nothing, the void type has to be declared as the return type, which can be omitted in JavaScript.
Class inheritance also works differently. For both JavaScript and C#, methods are implicitly final and can’t be overridden, unless the virtual keyword is added to the method declaration (for the two programming languages). The difference is that C# only overrides methods that contain the override keyword. On the other hand, JavaScript tries to override the class methods as soon as it inherits them, no override keyword needed. So, as an example of class inheritance for JavaScript, we will have:
class Weapon extends Item { //Class members and declarations }
The same code written in C# will be:
public class Weapon : Item { //Class members and declarations }
That’s the main differences between the two languages and it will practically define all others, such as yielding code execution, accessing GameObjects and components, raycasting, etc. There are other differences such as the keyword used to import libraries (“import” for JavaScript and “using” for C#) but these declaration and keyword differences are easily figured out.
So, if the reader understood these differences, all the other ones that will be explained on the next posts will make more sense.
One final last thing: even if you don’t have to declare the type of the variables and methods in JavaScript it is recommended that you do. This is due the fact that the compiler tries to figure out the type of variable to use, if it hasn’t been declared, which may lead to performance issues. e.g.:
//do this: private var score:int; //instead of this: private var score;
This wouldn’t be a issue if we were talking about code that runs every minute, but let’s not forget that games today runs practically at 60 fps, and Unity3D games are no exception. This way, add variable types whenever you can when programming JavaScript for Unity3D.
In the next post, differences between C# and JavaScript will be shown when programming a script that access other GameObjects and Components. You can read it here: Part 2 – GameObjects and Components.
Very interesting and useful posts!
I relay the series on my blog if you don’t mind.
Thanks! No problem.
Very good these tutorials, congratulations to the author!
Have been looking for someone to simply write this information out for ages, good job!