Unity3D: JavaScript vs. C# – Part 2
Posted by Dimitri | Nov 29th, 2010 | Filed under Programming
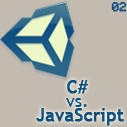
Part 2 of a post series that tries to explain the differences between JavaScript and C# when programming for the Unity3D game engine. It is recommended that you read part one before continuing. In this post, I will explain how to access other GameObjects and Components. This is one of he most common tasks that a programmer has to perform when writing scripts for Unity3D game engine. So, let’s start by assuming that we want to retrieve a GameObject named ‘Pawn’ which is at the root of the scene and has a script called ‘PawnMover’ attached to it.
Getting the GameObject using JavaScript is simple. All you have to do is to call the GameObject class Find() static method and pass the name of the other GameObject we want as a parameter:
private var pawnGO:GameObject; function Awake() { pawnGO = GameObject.Find("Pawn"); }
This is how you do it with JavaScript. With C#, it will be very similar:
using UnityEngine; using System.Collections; public class PawnGetter : MonoBehaviour { private GameObject pawnGO; void Awake () { pawnGO = GameObject.Find("Pawn"); } }
Without considering the two programming languages different notations and reserved keywords, the code is exactly the same (line 4 in the first block is the same as line 8 at the second). This makes sense, because it doesn’t matter if the code is strongly typed or weakly typed, GameObject.Find() method always returns a GameObject.
Now, let’s see how to get a Component inside a GameObject. Again, assuming the same ‘Pawn’ GameObject with a ‘PawnMover’ component attached to it, here is how to get the ‘PawnMover’ Component from another GameObject, using JavaScript:
private var pawnGO:GameObject; private var pmSC:PawnMover; function Awake() { pawnGO = GameObject.Find("Pawn"); pmSC = pawnGO.GetComponent("PawnMover"); }
Basically, to get the ‘PawnMover’ Component, all we needed to do is to call the GetComponent() method from the pawnGO GameObject and pass the desired component’s name as a parameter. Instead of the name, we could also have passed the type of the Component as the parameter but, for the above example, the name will do. This method returns a Component, and since JavaScript is weakly typed, we don’t have to cast the resulting Component to the PawnMover class. The same script in C# will be:
using UnityEngine; using System.Collections; public class PawnGetter : MonoBehaviour { private GameObject pawnGO; private PawnMover pmSC; void Awake() { pawnGO = GameObject.Find("Pawn"); //returns a CS0266 error pmSC = pawnGO.GetComponent("PawnMover");//<=returns a CS0266 error //this is the right way to do it when using C# pmSC = pawnGO.GetComponent< PawnMover >(); } }
With C#, it isn’t possible to just call the GetComponent() method and pass the component’s name as a parameter, since it causes a CS0266 error, meaning that C# can’t implicitly convert from one type another. That’s because C# is strongly typed, so we can’t convert from Component to PawnMover without a cast. That’s why we need to make a generic method call passing the type, thus, enforcing that the GetComponent() method returns a ‘PawnMover’ object instead of a Component.
That was a long post, but I hope there was something useful there. The next part of this series will explain some of the differences between JavaScript and C# when programming a script that moves a GameObject, which you can read here: Part 3 – Moving a GameObject.
But if in the c# code, i want to get another javascript , i can’t because c# can not deserialize the javascript into its internal class type.
pmSC = pawnGO.GetComponent(); this line will give error type or namespace PawnMover not found.
If you want to get a script written in JavaScript from C#, you will have to place it inside the Standard Assets folder. Maybe this post can be helpful: Unity3D: JavaScript->C# or C#->JavaScript access.
I am really SO excited to find someone who is describing how to go from Javascript TO C# rather than the other way round (which literally every result on Google gives you) but I still dont understand the BASIC reason why Javascript can do the code in the first picture on this page (javascript vs csharp2) in 5 lines when C# needs so much extra palaver…
I have learned to do it and made some stuff, but I still have NO idea why I NEED to? I feel like you are rushing over the explanation of why c# needs all the ‘using’ stuff, why everything needs to be in its own class etc.
As a person used to writing JS (kindof) I am REALLY confused by why UnityScript doesn’t need to do the Monobehaviour thing and so on, how does it work? Why does it work. I almost feel the C# makes more sense then the language I know…