Posts Tagged ‘Programming’
Programming related posts.
Unity3D: JavaScript vs. C# – Part 4
Posted by Dimitri | Filed under Featured, Programming
This is the 4th post of a series that tries to explain the main differences between JavaScript and C# when programming for Unity3D. In this post, some differences between yielding (pausing) code execution in these two programming languages will be pointed out. Before continuing, it is highly recommended that you read the first, second and third other posts of the series.
As explained before, yielding pauses the code execution, being very useful to game programming, as you have a better control when things will happen in your game. Whether using C# or JavaScript in Unity3D, one can’t simply yield the Update() method. There is a workaround for that, but as you might have guessed, they are different for the two programming languages we are discussing. Since these workarounds are often used, they will be the examples presented on this post. Let’s start by seeing how to yield a JavaScript code: (more…)
Unity3D: JavaScript vs. C# – Part 3
Posted by Dimitri | Filed under Programming
This is the third part of a series that show some of the differences between JavaScript and C# when writing scripts for Unity3D game engine. I suggest that you read the first and second post of the series to better understand what is going on here.
In this third part, I will point out some differences between JavaScript and C# by writing a script that makes a GameObject move forward. So, let’s start with the programming language that will take the smallest number of lines to make a GameObject move, JavaScript: (more…)
Unity3D: JavaScript vs. C# – Part 2
Posted by Dimitri | Filed under Programming
Part 2 of a post series that tries to explain the differences between JavaScript and C# when programming for the Unity3D game engine. It is recommended that you read part one before continuing. In this post, I will explain how to access other GameObjects and Components. This is one of he most common tasks that a programmer has to perform when writing scripts for Unity3D game engine. So, let’s start by assuming that we want to retrieve a GameObject named ‘Pawn’ which is at the root of the scene and has a script called ‘PawnMover’ attached to it.
Getting the GameObject using JavaScript is simple. All you have to do is to call the GameObject class Find() static method and pass the name of the other GameObject we want as a parameter: (more…)
Android OpenGL: Get the ModelView Matrix on Cupcake (1.5)
Posted by Dimitri | Filed under Programming
One of the most annoying things when developing apps with OpenGL in Android 1.5, is the fact that there’s no access to the ModelView or the Projection matrices. It is not possible to call the glGetFloatv function because it wasn’t implemented until OpenGL ES 1.1, which isn’t available in the Cupcake versions. So, how to get the ModelView matrix on Android 1.5? The first thing you are going to do is grab these three classes: MatrixGrabber, MatrixStack and MatrixTrackingGL. All of them are inside the API demos, under the package com.example.android.apis.graphics.spritetext.
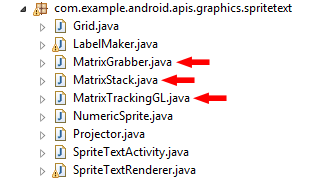
You're going to need these.
Unity3D: JavaScript vs. C# – Part 1
Posted by Dimitri | Filed under Featured, Programming
This is the first post of a series that will discuss some differences between JavaScript and C# when programming for Unity3D game engine. A project with all the scripts used in these posts will be available for download in the last post of the series. So let’s start from the start and see some of the main differences between the two languages.
The first and most easily distinguishable is the notation used to declare variables and methods. When programming in JavaScript, it isn’t necessary to declare the variable or method type whether in C#, it’s a must. That is because JavaScript is a weakly typed language, meaning that the interpreter chooses the best type to use at compilation-time. C# is a totally different because it is strongly typed, so, the type has to be declared before a variable or method. Let’s see some examples to better grasp this concept. The following script is a JavaScript example: (more…)