Android: How to return RGB values from an image file
Posted by Dimitri | Apr 21st, 2011 | Filed under Programming
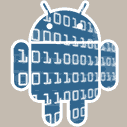
Surprisingly, it’s quite easy to get the RGB value from an image file in Android. It’s certainly a lot easier than retrieving the pixel values from the Camera Preview. All that is required is to load the image file into a Bitmap object and them call the getPixel() method from the loaded bitmap.
It’s also possible to call this method to create an array of alpha and RGB values (ARGB) without calling the copyPixelsToBuffer() method, avoiding the use of buffers in Android which make things more complicated than it should be. As an example, the following code prints the color values of the pixels from the 4 corners of the image in LogCat and creates a RGBA array that stores each pixel color value:
package fortyonepost.com.iapa; import android.app.Activity; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.os.Bundle; import android.util.Log; public class ImageAsPixelArray extends Activity { //a Bitmap that will act as a handle to the image private Bitmap bmp; //an integer array that will store ARGB pixel values private int[][] rgbValues; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //load the image and use the bmp object to access it bmp = BitmapFactory.decodeResource(getResources(), R.drawable.four_colors); //define the array size rgbValues = new int[bmp.getWidth()][bmp.getHeight()]; //Print in LogCat's console each of one the RGB and alpha values from the 4 corners of the image //Top Left Log.i("Pixel Value", "Top Left pixel: " + Integer.toHexString(bmp.getPixel(0, 0))); //Top Right Log.i("Pixel Value", "Top Right pixel: " + Integer.toHexString(bmp.getPixel(31, 0))); //Bottom Left Log.i("Pixel Value", "Bottom Left pixel: " + Integer.toHexString(bmp.getPixel(0, 31))); //Bottom Right Log.i("Pixel Value", "Bottom Right pixel: " + Integer.toHexString(bmp.getPixel(31, 31))); //get the ARGB value from each pixel of the image and store it into the array for(int i=0; i < bmp.getWidth(); i++) { for(int j=0; j < bmp.getHeight(); j++) { //This is a great opportunity to filter the ARGB values rgbValues[i][j] = bmp.getPixel(i, j); } } //Do something with the ARGB value array } }
There isn’t much to say about this code except that the a Bitmap object was created at line 12 and line 15 creates a 2 dimensional Integer array to store the pixels from the image. The image is loaded at line 25 and right after that, the size of the array is defined (line 28). Then, lines 32 through 38 output the value of each one of the pixels at the four corners of the image. Note that the getPixel() method requires two parameters: the X and Y coordinates of the pixel inside the image. These coordinates are the usual ones when working with image files: their axis goes from left to right and top to bottom.
Another detail is that the aforementioned method returns hexadecimal Integer values. That’s the reason why they are being converted with the toHexString() method before getting outputted to LogCat. This hexadecimal integer is composed by four value pairs that range from 00 to FF. The first two correspond to the alpha value of the image, where 00 is transparent and FF is completely opaque. The other three pairs determine the red, green and blue values, in that particular order.
Finally, the rgbValues array is filled with pixel color values (from line 41 to 48).
So, if this image is loaded by the above code:

It would output the following:
Top Left pixel: ffff0000 Top Right pixel: ff00ff00 Bottom Left pixel: ff0000ff Bottom Right pixel: ffffffff
Comments? Suggestions? Please use the Comment field!
Hi, I’m trying to implement your code but i cant seem to figure out what four_colors is. sorry if it’s obvious but im really new into android developing.
Thanks a lot in advance for your help!
nvm i got it figured ^^ sorry for wasting your time
…i can’t figure it out, can you help and advice what and how to set this value please?