Android: obtaining SD card memory information
Posted by Dimitri | Sep 27th, 2011 | Filed under Programming
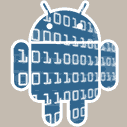
This Android tutorial explains how to obtain the primary external storage (normally, the SD card) information, such as the total available space and how much memory it has left to be consumed by applications and data. Fortunately, this is a short and simple code, that can be used to create file explorer app, or to check if the SD card has enough space to copy data into the external storage. An example Eclipse project with the source code is available for download at the end of the post. It requires Android 2.1 (Eclair) and works both on a real and emulated devices.
In simple terms, the code below checks for a connected SD card, by querying its state. If a external device was found, it then obtains the external memory’s total and available sizes, returning the values in GB, MB, KB and bytes:
package fortyonepost.com; import java.text.NumberFormat; import android.app.Activity; import android.graphics.Color; import android.os.Bundle; import android.os.Environment; import android.os.StatFs; import android.widget.TextView; public class SDcardInfoActivity extends Activity { //the statistics of the SD card private StatFs stats; //the state of the external storage private String externalStorageState; //the total size of the SD card private double totalSize; //the available free space private double freeSpace; //a String to store the SD card information private String outputInfo; //a TextView to output the SD card state private TextView tv_state; //a TextView to output the SD card information private TextView tv_info; //set the number format output private NumberFormat numberFormat; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //initialize the Text Views with the data at the main.xml file tv_state = (TextView)findViewById(R.id.state); tv_info = (TextView)findViewById(R.id.info); //get external storage (SD card) state externalStorageState = Environment.getExternalStorageState(); //checks if the SD card is attached to the Android device if(externalStorageState.equals(Environment.MEDIA_MOUNTED) || externalStorageState.equals(Environment.MEDIA_UNMOUNTED) || externalStorageState.equals(Environment.MEDIA_MOUNTED_READ_ONLY)) { //obtain the stats from the root of the SD card. stats = new StatFs(Environment.getExternalStorageDirectory().getPath()); //Add 'Total Size' to the output string: outputInfo = "\nTotal Size:\n"; //total usable size totalSize = stats.getBlockCount() * stats.getBlockSize(); //initialize the NumberFormat object numberFormat = NumberFormat.getInstance(); //disable grouping numberFormat.setGroupingUsed(false); //display numbers with two decimal places numberFormat.setMaximumFractionDigits(2); //Output the SD card's total size in gigabytes, megabytes, kilobytes and bytes outputInfo += "Size in gigabytes: " + numberFormat.format((totalSize / (double)1073741824)) + " GB \n" + "Size in megabytes: " + numberFormat.format((totalSize / (double)1048576)) + " MB \n" + "Size in kilobytes: " + numberFormat.format((totalSize / (double)1024)) + " KB \n" + "Size in bytes: " + numberFormat.format(totalSize) + " B \n"; //Add 'Remaining Space' to the output string: outputInfo += "\nRemaining Space:\n"; //available free space freeSpace = stats.getAvailableBlocks() * stats.getBlockSize(); //Output the SD card's available free space in gigabytes, megabytes, kilobytes and bytes outputInfo += "Size in gigabytes: " + numberFormat.format((freeSpace / (double)1073741824)) + " GB \n" + "Size in megabytes: " + numberFormat.format((freeSpace / (double)1048576)) + " MB \n" + "Size in kilobytes: " + numberFormat.format((freeSpace / (double)1024)) + " KB \n" + "Size in bytes: " + numberFormat.format(freeSpace) + " B \n"; //output the SD card state tv_state.setTextColor(Color.GREEN); tv_state.setText("SD card found! SD card is " + externalStorageState +"."); //output the SD card info tv_info.setText(outputInfo); } else //external storage not found { //output the SD card state tv_state.setTextColor(Color.RED); tv_state.setText("SD card not found! SD card state is \"" + externalStorageState + "\"."); } } }
At the beginning of the above Activity, there are eight variables being declared. The first is a StatFs variable that stores information about the external storage (line 15). After that, there’s the String externalStorageState, which is later used to flag the external state (line 17).
Moving on, the two doubles are going to store the total memory of the external storage and the memory left that can be used by applications (lines 20 and 22). The purpose of the other declared variables at the beginning of the code is to display the information at the screen, so there is a String, two TextView objects and a NumberFormat object (lines 25 through 32).
At the onCreate() function, the TextView objects are initialized (lines 42 and 43) so as the externalStorageState, with the String returned by the Environment.getExternalStorageState() method, which returns the primary external storage state, such as mounted, unmounted, read only, etc (line 46). The obtained state is checked at the if statement, right below it (lines 49 through 51), checking all states where the SD card is present and the memory usage information is retrievable.
Case the external storage is available, the stats object is initialized, passing the external memory root path as a parameter to the StatFs constructor (line 54). The stats object will store all the information about the SD card usage. Then, at line 60, the total size of the external storage is calculated by multiplying the total number blocks with the default block size (in bytes). This value is stored at the totalSize double.
Lines 63 through 67 are the ones that set the number format of the output String, to only display the first two decimal characters. That way, it’s more more easy to understand the numbers that this application outputs. As an example printing the memory value as 1.24 GB is more intuitive than showing it as 1.33218304E9 GB.
Now, the obtained total memory of the external storage must be displayed. Since the totalValue is the total memory in bytes, a conversion must be made to display it in gigabytes, megabytes and kilobytes. The values used for the conversion can be obtained here. After the conversion, the values are added to the outputInfo String (lines 70 through 73). The same process is applied at line 80, to determine the remaining free space at the external storage, however, instead of multiplying the total number blocks with the default block size, the number of available blocks is multiplied with the total number of blocks. This value is stored at the freeSpace double (line 79).
Continuing with the code, after calculating the remaining space, the same process is done to the freeSpace variable: the freeSpace is converted in GB, MB and KB values with a simple division and is converted to a String and stored in the outputInfo (lines 82 through 85). With all values obtained, the last thing to do is to display them using the tv_info variable (line 92). Not only that, but the status of the external memory is also displayed with a TextView (line 89). Case the if statement at line 49 returns false, the else block will execute, displaying an error message in red with the current state of the SD card, through the tv_state TextView.
Here’s an screenshot of this application:
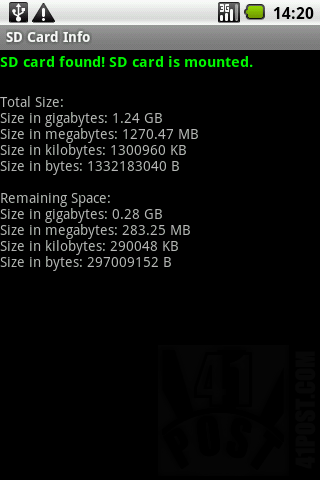
This is what the example application looks like.
Don’t forget to download it here:
Hey nice explanation ..
Its very helpful for me…
thanks
i make one program that give us the sd card information so this code will help me but i also want to see the content of the sd card
therefore reply me if you have any solution for that………!!!!!!!!!!!
Thanks
Tejas rana