Android: Acessing the gyroscope sensor for simple applications
Posted by Dimitri | Apr 27th, 2011 | Filed under Programming
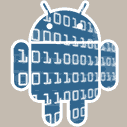
This post explains how to get values from the gyroscope (or any sensor that returns the device’s relative angle) to create simple application. The reason why I’m stating ‘for simple applications’ is because the code featured here is already deprecated. I’m just explaining how to do it because it still works, and it’s very clean and short to explain, as opposed to the new method, which is much more accurate but more complex to implement.
Still, it’s possible to use it for simple applications, although, if the application requires accuracy from the sensors, such as augmented reality applications or even games, it’s recommended to use the getRotationMatrix() method from the Sensor Manger class instead.
With that said, the following code just prints the rotation values from the gyroscope on the screen:
- package fortyonepost.com.ag;
- import android.app.Activity;
- import android.hardware.Sensor;
- import android.hardware.SensorEvent;
- import android.hardware.SensorEventListener;
- import android.hardware.SensorManager;
- import android.os.Bundle;
- import android.widget.TextView;
- public class AccessGyroscope extends Activity implements SensorEventListener
- {
- //a TextView
- private TextView tv;
- //the Sensor Manager
- private SensorManager sManager;
- /** Called when the activity is first created. */
- @Override
- public void onCreate(Bundle savedInstanceState)
- {
- super.onCreate(savedInstanceState);
- setContentView(R.layout.main);
- //get the TextView from the layout file
- tv = (TextView) findViewById(R.id.tv);
- //get a hook to the sensor service
- sManager = (SensorManager) getSystemService(SENSOR_SERVICE);
- }
- //when this Activity starts
- @Override
- protected void onResume()
- {
- super.onResume();
- /*register the sensor listener to listen to the gyroscope sensor, use the
- callbacks defined in this class, and gather the sensor information as quick
- as possible*/
- sManager.registerListener(this, sManager.getDefaultSensor(Sensor.TYPE_ORIENTATION),SensorManager.SENSOR_DELAY_FASTEST);
- }
- //When this Activity isn't visible anymore
- @Override
- protected void onStop()
- {
- //unregister the sensor listener
- sManager.unregisterListener(this);
- super.onStop();
- }
- @Override
- public void onAccuracyChanged(Sensor arg0, int arg1)
- {
- //Do nothing.
- }
- @Override
- public void onSensorChanged(SensorEvent event)
- {
- //if sensor is unreliable, return void
- if (event.accuracy == SensorManager.SENSOR_STATUS_UNRELIABLE)
- {
- return;
- }
- //else it will output the Roll, Pitch and Yawn values
- tv.setText("Orientation X (Roll) :"+ Float.toString(event.values[2]) +"\n"+
- "Orientation Y (Pitch) :"+ Float.toString(event.values[1]) +"\n"+
- "Orientation Z (Yaw) :"+ Float.toString(event.values[0]));
- }
- }
Let’s take a closer look at the code. Right at the start, it declares a TextView and a SensorManager object (lines 14 and 16). These are going to be used to output the values to the screen and to store a handle to the gyroscope sensor. The onCreate method initializes these two variables, getting the TextView from the main.xml file and the returning a reference to the system’s sensors (lines 26 and 29).
The onResume() method runs every time the Activity starts its lifecycle and inside it, line 40 defines three different pieces of information: the first parameter defines that sManager (the sensor manager handle) should use the sensor event listener callbacks defined below. The second parameter sets what kind of sensor the sensor manager will retrieve values from. The third and last one defines the rate at which the sensor’s data must be gathered.
Next the code inside the onStop() method unregisters all the callbacks we have set, since it is called when the application is no longer visible (line 48). The methods onAccuracyChanged() and onSensorChanged() are being declared because the class is implementing the SensorEventListener interface, which allows the definition of the sensor callbacks.
That means that it’s possible to execute a code every time the values from a sensor change or when the accuracy of the information returned by a sensor changes. In this example, the accuracy doesn’t matter, so the implementation of this callback is left blank (line 53 to 56). However the onSensorChanged() method is going to have some code inside it, since we want it to print the values obtained by the sensors every time they change.
Inside the onSensorChanged(), the if statement tests whether the information of the sensor can be relied on. If true, the method returns void (lines 62 to 66) and no further code is executed. After checking this, we print out the values obtained by the gyroscope in the tv (TextView) object. These values are returned as an array that is a member of the event object. Note that different sensors will return different values, and event.values[0] is not always going to be the Yaw. For more information, read this page for a reference on the possible returned values.
Again, the code shown here is already deprecated and should be used only for simple interactions between the sensors and the software or preferably, for testing purposes.
Download
Here’s a source Eclipse project with the code featured in this post:
This is not gyroscope example this code above is not using gyroscope sensor.It is using orientation sensor at the android phone.
dude, orientation sensor that u mentioned is the gyroscope in the android phone.
This is Acelerometer, but not gyroscope