Android: changing the screen brightness
Posted by Dimitri | Mar 28th, 2011 | Filed under Programming
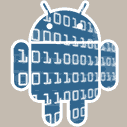
This post will explain how to change the current system brightness with a seek bar GUI on Android devices. The code here featured only works on real devices, because it is not possible to see brightness changes on the emulator. Also, all the code explained here is available for download at the end of this post.
The first thing one must know is that Android system brightness value is applied to the screen’s backlight only when the screen turns on. This means that only after a boot up or awaking the phone from a sleeping state will make the screen as bright as the value defined by the System.SCREEN_BRIGHTNESS variable. Consequently, changing only that variable won’t be enough to preview the brightness level.
That’s why it’s necessary to access the current window brightness by setting the screenBrightness property located at the the LayoutParams object that is obtained from the current window. Then, we use the same value to set the system brightness and window brightness, so it can be previewed before its set.
Here’s the code:
package fortyonepost.com; import android.app.Activity; import android.content.ContentResolver; import android.os.Bundle; import android.provider.Settings.SettingNotFoundException; import android.provider.Settings.System; import android.util.Log; import android.view.Window; import android.view.WindowManager.LayoutParams; import android.widget.SeekBar; import android.widget.SeekBar.OnSeekBarChangeListener; public class BrightnessActivity extends Activity { //UI objects// //the seek bar variable private SeekBar brightbar; //a variable to store the system brightness private int brightness; //the content resolver used as a handle to the system's settings private ContentResolver cResolver; //a window object, that will store a reference to the current window private Window window; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //get the seek bar from main.xml file brightbar = (SeekBar) findViewById(R.id.sb_brightbar); //get the content resolver cResolver = getContentResolver(); //get the current window window = getWindow(); //seek bar settings// //sets the range between 0 and 255 brightbar.setMax(255); //set the seek bar progress to 1 brightbar.setKeyProgressIncrement(1); try { //get the current system brightness brightness = System.getInt(cResolver, System.SCREEN_BRIGHTNESS); } catch (SettingNotFoundException e) { //throw an error case it couldn't be retrieved Log.e("Error", "Cannot access system brightness"); e.printStackTrace(); } //sets the progress of the seek bar based on the system's brightness brightbar.setProgress(brightness); //register OnSeekBarChangeListener, so it can actually change values brightbar.setOnSeekBarChangeListener(new OnSeekBarChangeListener() { @Override public void onStopTrackingTouch(SeekBar seekBar) { //set the system brightness using the brightness variable value System.putInt(cResolver, System.SCREEN_BRIGHTNESS, brightness); //preview brightness changes at this window //get the current window attributes LayoutParams layoutpars = window.getAttributes(); //set the brightness of this window layoutpars.screenBrightness = brightness / (float)255; //apply attribute changes to this window window.setAttributes(layoutpars); } @Override public void onStartTrackingTouch(SeekBar seekBar) { } @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { //sets the minimal brightness level //if seek bar is 20 or any value below if(progress<=20) { //set the brightness to 20 brightness=20; } else //brightness is greater than 20 { //sets brightness variable based on the progress bar brightness = progress; } } }); } }
The above code works by setting a variable to get the values returned by the seek bar defined at the main.xml file (line 18). Then, other three variables are created to store the system brightness, the application's content and the current windows (lines 21, 23 and 25) respectively. After initializing these variables (line 35, 38 and 41), some seek bar's settings are set, like the value range and value increment (lines 45 and 47).
The max value was set to 255 because the system brightness is defined by a value in the 0 – 255 range. The next lines contain a try/catch block that tries to access the current system brightness and store it at the brightness variable (lines 49 to 59). Based on this value, the seek bar progress is set at line 62.
Next, the brightbar listeners are set so that this seek bar can actually change the values based on user input. The last method of this interface, onProgressChange() (line 88) is called when the seek bar value is changed by the user. There, we have a simple if statement that assigns the progress value to the brightness variable and avoids letting the brightness to be set below 20, because, in some devices, anything below that value would actually turn off the screen! If that happens to you don't panic and DO NOT hit the back button, just try to scroll the seek bar forward.
The first method of the OnSeekBarChangeListener implementation (line 68) is called when the user releases the seek bar, and it's where both window and system brightness are set. At line 71, the system brightness is finally set to have the same value as the variable brightness. Line 75 returns the current window layout parameters as a object. With that, the brightness of the window is set (line 77). Since brightness from the window layout parameters is set with a float in the 0 – 1 range, the brightness variable is divided by 255 cast as a float, this way, it will return a value with decimal places. Line 79 finally apply all the changes made to the layoutpars object to the current window, making it have the same brightness as the system brightness.
Lastly, add the WRITE permission to the Manifest file:
<uses-permission android:name="android.permission.WRITE_SETTINGS"></uses-permission>
That's it!
This was very useful, thank you.
Thanks!
Thanks, this is exactly what I was looking for!
Doesn’t working on ICS :(
after quit this activity, the setting is gone! how to change the brightness and make it’s enable all the time.