Game Programming Basics: Creating a FPS counter
Posted by Dimitri | Feb 18th, 2011 | Filed under Programming
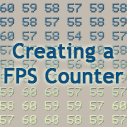
Sometimes, while creating a game, a programmer realizes that he/she needs to make sure if some part of the code is running fast enough, before adding more things that could cause the game to slowdown. To properly measure a game’s performance, there is the need to program a FPS (frames per second) counter. As the name suggests, it will count the number of frames that where rendered at the period of one second.
This is an essential information when creating games, as it will serve as a strong indicator to measure the performance impact of a recently added element to the game.
Here’s the code for a FPS counter, written in Java:
final public class FPScounter { private static int startTime; private static int endTime; private static int frameTimes = 0; private static short frames = 0; /** Start counting the fps**/ public final static void StartCounter() { //get the current time startTime = (int) System.currentTimeMillis(); } /**stop counting the fps and display it at the console*/ public final static void StopAndPost() { //get the current time endTime = (int) System.currentTimeMillis(); //the difference between start and end times frameTimes = frameTimes + endTime - startTime; //count one frame ++frames; //if the difference is greater than 1 second (or 1000ms) post the results if(frameTimes >= 1000) { //post results at the console Log.i("FPS", Long.toString(frames)); //reset time differences and number of counted frames frames = 0; frameTimes = 0; } } }
The explanation on how the above code works will make more sense after you see how to use this class. To have a correct FPS reading, you will need to know exactly how your game loop is set up. Then call the StartCounter() and the StopAndPost() methods, at the beginning and at the end of the game loop, like this:
//start counting FPS FPScounter.startCounter(); //your game loop Physics(); Update(); Draw(); //Stop counting, and post the results FPScounter.StopAndPost();
With that said, the FPSCounter code works by getting the current system time in the beginning of the game loop with the startCounter() method. This method gets system time and stores it at the startTime variable (line 12). Then, after the whole game loop is complete, call the StopAndPost() method. Inside it, the current system time is stored at the endTime variable (line 19).
After that, the difference between the system times when the first and the second methods of this class were called is calculated, and stored in the frameTimes variable. It is necessary to know the difference because there is no guarantee that all frames will be called at a constant rate. Add this with other existing time differences by adding the frameTime value to itself (line 21).
Finally, the frames variable is incremented by one (line 23), counting the number frames. The last thing that this method does is to check if the time differences exceeded 1000 milliseconds (or 1 second). If it does, print the current FPS (line 28). The following lines, resets the values of the frame counter (frames variable) and the difference between frame times (frameTimes variable) to zero, so the class can calculate the current FPS all over again.
Observations
As anything that is added to a program, the code explained on this post will take some of the application resources. In case it starts to make your game lag, try to print FPS at longer intervals, such as every 3 seconds, instead of every second:
//if the difference is greater than 3 seconds (or 3000ms) post the results if(frameTimes >= 3000) { //post results at the console Log.i("FPS", Long.toString(frames/3)); //reset time differences and number of counted frames frames = 0; frameTimes = 0; }
It is essential to know how your game loop was set up, if some portion of its code uses a different thread and the way the your graphics library works. These factors have an influence on this code, making it print values that do not correspond to the real FPS. This code isn’t the only answer to find out a game’s FPS, and depending on how the game loop was written, it is going to be required that you divide or multiply the frames variable with a fixed value to make it work correctly.
And that’s it!
this fps is good for android games too ? can I use the fps to counter fps for android games?
Yes, you can use it for Android games, but you will have to adjust the output of the frames variable, which may not show the correct FPS value. This adjustment depends heavily whether you are using the Canvas or the OpenGL to render your game, the method you used to set up your game loop and whether the game is running from an emulated or real device.
Yeah you can use this in an Android application.
if sbd want to use it constantly replace original if statement with following:
if(frameTimes >= 1000)
{
//post results at the console
Log.i(“FPS”, Long.toString(frames));
//reset time differences and number of counted frames
frames = 0;
frameTimes = 0;
startTime = endTime;
}
Errata (track frames in f.e. OPENGL)
whole fc:
public final static void StopAndPost()
{
//get the current time
endTime = (int) System.currentTimeMillis();
//the difference between start and end times
//frameTimes = frameTimes + endTime – startTime;
frameTimes = endTime – startTime;
//count one frame
++frames;
//if the difference is greater than 1 second (or 1000ms) post the results
if(frameTimes >= 1000)
{
//post results at the console
Log.i(“FPS”, Long.toString(frames));
//reset time differences and number of counted frames
frames = 0;
frameTimes = 0;
startTime = endTime;
}
}