Android: Loading and playing videos from different sources
Posted by Dimitri | Feb 15th, 2011 | Filed under Featured, Programming
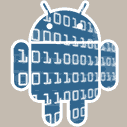
This is the 41st post on the website! It explains how to load images from a variety of locations, such as the ‘Resources’ folder, the SD card and from a remote server. As usual, the source code is available for download at the end of the post.
Let’s start with the basics: video formats. At the time this is being written, Android supports .3gp and .mp4 video files encoded as H.263,H.264 and MPEG-4 SP file formats. For an updated list, visit this link.
To load and play videos, we are going to use Android’s VideoView class. The easiest way to do that in Eclipse, is to use the interface editor. Just select the main.xml file and then, drag and drop a VideoView element inside the screen. After that, go to the Properties tab and give it an ID that will be used later to identify the VideoView at the code.
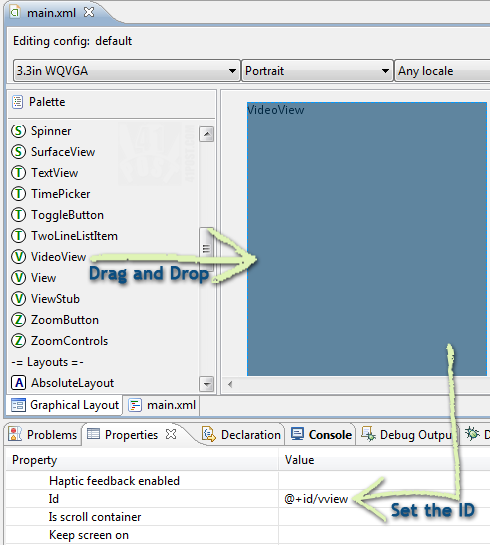
This is how to add the VideoView to the application's interface.
The code to play videos on Android using the VideoView class will always be the same, what differs is the part that loads the videos from a given source. To avoid repeating the same code over and over throughout the post, here’s the basic structure that plays a video file, without the loading part:
/*Imports and package declarations omited*/ public class PlayVideo extends Activity { private VideoView vView; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { //sets the Bundle super.onCreate(savedInstanceState); //sets the context setContentView(R.layout.main); //get the VideoView from the layout file vView = (VideoView)findViewById(R.id.vview); //use this to get touch events vView.requestFocus(); /*This is where you put the code that loads *the video file*/ //... //enable this if you want to enable video controllers, such as pause and forward vView.setMediaController(new MediaController(this)); //plays the movie vView.start(); } }
Here’s how it works: a VideoView variable (vView) is declared at the fourth line. At the moment the Activity is created, the VideoView object is initialized with the data contained at the layout.xml file (line 16).
After that, the Activity requests focus, so the VideoView object can respond to touch events. Then, there’s the part where the video is loaded, which will be explained at the next sections of this post. Lastly, the method setMediaController() is called to display the Play, Pause and Seek buttons at the bottom of the video. This line isn’t required, and you can leave the code without it case you want to hide the playback options from the user. Line 29 sets the video to play when this Activity is launched. Again, this isn’t necessary to make it work, and case you want the video to start paused, simply don’t add this line.
This is how the Activity needs to be set up with the VideoView object to play the video files, now for the loading part…
Loading Videos from the SD card
To load videos from the SD card, we need to put a video file inside it. To add the video file to a SD card of an emulated device, go to the DDMS perspective in Eclipse, a device at the the “Devices” tab, find the ‘sdcard’ folder and then, press the Push file into the device button.
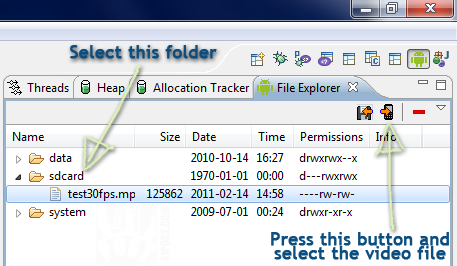
After selecting the device, find the 'sdcard' folder and click the 'Push file into the device' button, as shown.
Just select the video file at the next dialog. Now, to load the video from the SD card and play it, we need to call the setVideoPath method of the vView object, like this:
//load video named "test30fps" video from the root of the SD card vView.setVideoPath("/sdcard/test30fps.mp4");
Replace line 23 in the code at the beginning of the post with the above.
Since the code is going to access the SD card, it is necessary to add a permission to the Android Manifest file:
<uses-permission android:name="android.permission.STORAGE"></uses-permission>
Add this line right after the one that contains the android:versionName on it.
Loading images from a the ‘Resources’ folder or a remote server
To load videos from the ‘Resources’ (‘res’) folder, we need to have a video file inside it, at the raw subfolder. If this folder isn’t already there, you must create it. This can be accomplished in Eclipse, by right clicking the res folder and then selecting: New->Folder. Then just drag and drop the video file in there. You will end up with something like this:
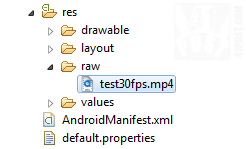
The video file has to be placed inside the 'raw' folder.
The method required to load a video from the Resources folder or from a remote location is going to be different – we can’t simply call the setVideoPath() like before. The video is going to be passed as an URI (Uniform Resource Identifier), which is an address to an internet resource. For that, we need to declare a String variable at the beginning of the code, to store the path of the file. Instead, the method that needs be used is the setVideoURI(), which takes an URI as a parameter. So, here’s the code to load a video from the Resources folder.
public class PlayVideo extends Activity { //creates a string to store the video location private String vSource; @Override public void onCreate(Bundle savedInstanceState) { //... //replace line 23 with these lines of code //loads video from the Resources folder //set the video path vSource ="android.resource://fortyonepost.com.vv/" + R.raw.test30fps; //set the video URI, passing the vSourse as a URI vView.setVideoURI(Uri.parse(vSource)); //... } }
To do it from a remote server, a real Android device is going to be required, because loading a video from a remote server doesn’t work on an emulated device. And finally, here’s the code to load a video from a remote server:
public class PlayVideo extends Activity { //creates a string to store the video location private String vSource; @Override public void onCreate(Bundle savedInstanceState) { //... //replace line 23 with these lines of code //loads video from remote server //set the video path vSource ="rtsp://v6.cache1.c.youtube.com/CjYLENy73wIaLQnF4qJzpSt4nhMYESARFEIJbXYtZ29vZ2xlSARSBXdhdGNoYMDFmvL1wMysTQw=/0/0/0/video.3gp"; //set the video URI, passing the vSourse as a URI vView.setVideoURI(Uri.parse(vSource)); //... } }
Don’t forget to add the following line to the Manifest file of the app, so it can access the Internet:
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
Add this line right after the one that contains the android:versionName on it.
Note: it is also possible to use URIs to load video files from the SD card. The process is exactly the same, what will change is the path used to create the URI (it must point to the SD card).
Here’s the source code:
Download
thanks..
To add the video file to a SD card of an emulated device..when i press the Push file into the device button.it shows an
error:Failed to push selection: Read-only file system
so please give me suggestions….
I have never seen this happen before. Are you trying to push the file into the root of the SD card? Maybe the folder you are trying to push your video file into doesn’t have the write (w) permission. Under the File Explorer tab, find the Permissions column. What are the permissions there? Is your directory marked as d–rwxr-x?
this code works perfectly…
but when i install apk on emulator….i am geeting this message “sorry,this video cannot played”…
please anyone can helps!
Be sure that you are writing the path right, “android.resource://com.yourpackage/”, i got the same message until i fix that
thanks for this kind of tutorial. keep it up man……..
It says there are 3 errors with the script:
setContentView(R.layout.main); main cannot be resolved or is not a field
(new MediaController(this)); MediaController cannot be resolved to a type
vView = (VideoView)findViewById(R.id.vview); vview cannot be resolved or is not a field
HELP!
Maybe you need to add the following import:
import android.widget.MediaController;
That should solve the second error. As for the other two, have you added a VideoView to the main.xml file? If not, do it and name it vview. Also, define the package your class is using by typing package plus the name of the package your Activity was placed into, something like: package com.something.example.
If you have already done that, and the errors are still appearing, try this:
– Delete the auto generated R class (inside the “gen” folder)
– On Eclipse select the project folder, and then click on Project->Clean…
Well, I used this to load a video from the resources, and everything works until the video start playing. when I click start on the controller, the video starts, but at the same time I get the “the application has ended unexpectadly” message, and the application crashes.
Great tutorial. In the DDMS i get an error says “buffer count(4) is too low. increase to 12” and the “sorry video cant be displayed.” please help.
i m getting the same problem… buffer count(4) is too smal increased to 12……any answer u get..
When I am trying to give out the url like youtube.com videos it is saying that sorry video cannot be displayed, can i know the reason and wt to be included inorder to play those videos… thank you
The regular Youtube URLs can’t be used with the code featured on this tutorial, because the videos must be encoded on the 3gp format (or any other format compatible with the VideoView). Take a look at the video URL in the PlayVideoRemote Activity.
The URL there was obtained from http://m.youtube.com, and not from http://www.youtube.com.
Hey! Nice tutorial… but I’m having a small problem. I hope you can help me out!
Everything seems to be fine but when the video runs I can only hear the audio and I see a black screen.. The rest is fine, I can even see the media controllers!
That’s weird. I have no idea what could be causing this. Where was the app being executed? On a real or at an emulated device? Has anyone else experienced the same problem?
it will not playing also your link it shows sorry,this video cannot be played.
I get an error message saying the video cannot be played :(
For everyone having problems playing the videos from the SD card using the example project, please keep in mind that the video file must be placed on the root of the SD card before trying to play it. The example app won’t copy the video to the card by itself. Also, be sure to check this comment from Yotes, to make sure the path to the video file is the correct one.
Hi, thanks for the tutorial! Its cool. But i got a problem, everything work perfectly. But my video is not showing, however the background music of my video still can be hear. Just except the video wont appear. Has anyone else experienced the same problem? Please help me :(
Test your application on any mobile, it’ll work fine…..sometimes it does not work on emulator.
Test your application on any mobile, it’ll work fine…..sometimes it does not work on emulator.
Can any one tell how to play sever side videos…
i play the video through this video but i am facing the problem that’s video will not show only audio comes. how to recitifiy this problem as soon as possible my video format is 3gp
plz can any one tell me how to develop live streaming tv in android
i wanna code for live streaming tv in android
Thanks for this tutorial,nice one.
hi
hye…i wanna ask…can it loading video from apps like tiny cam monitor app?