Unity3D: JavaScript->C# or C#->JavaScript access
Posted by Dimitri | Dec 18th, 2010 | Filed under Featured, Programming
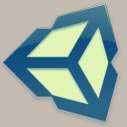
When programming for Unity3D, there are some cases where we need to access a script written in another programming language that isn’t the one we are currently using. Although it is highly recommended to convert all scripts to the same one, it is useful to know how to access a C# script from a JavaScript class and the other way around.
The first thing to do is place the scripts in the correct folders in your Project tab. The script you want to have access must be inside the Standard Assets or the Plugins folder. The other script has to be placed outside these folders. After this step, just call the GetComponent() method as any other Component. Here’s a JavaScript example:
//create a variable to access the C# script private var csScript : CSharp1; function Awake() { //Get the CSharp Script csScript = this.GetComponent("CSharp1"); //Don't forget to place the 'CSharp1' file inside the 'Standard Assets' folder } //...
Now, here’s a C# example:
using UnityEngine; using System.Collections; public class CSharp2 : MonoBehaviour { //create a variable to access the JavaScript script private JS1 jsScript; void Awake() { //Get the JavaScript component jsScript = this.GetComponent<JS1>(); //Don't forget to place the 'JS1' file inside the 'Standard Assets' folder } //... }
And this is how it’s done. There’s no way to have access to C# and JavaScript simultaneously, as one of the scripts must be at Standard Assets or Plugins folder. Scripts placed inside one of these folders are compiled first, meaning these scripts can’t access the ones outside it, as they are not compiled yet (more information here).
I have put together a project with examples, which you can download by clicking at the following link:
As usual, comments are welcome.
Thanks.. great job. It really helped.
…Liven… (ashish)
I need to access a C# script from a JavaScript class.
Try your .zip file, here is error message:
NullReferenceException: Object reference not set to an instance of an object
when run
GUI.Label(new Rect(10,30,500,20), csScript.message);
Anyone has same error or knows what do I miss here?
Which Unity version are you using?
I’ve downloaded and tested this project on the newest Unity version (3.4) and everything worked as expected.
Maybe you could recompile the scripts?
Got the same issue.
The problems cames that the script must be added to a gameObject. Leaving it in the project without affecting it to a gameObject will create a “NullreferenceException”
nice work, it really helped me!
ok I thought this would fix my problem but I get this error
Object reference not set to an instance of an object
I have a public bool variable in C# which is located in standard asset folder. the java script in trying to access this variable and change it to false or true. but as I said before I get that error when trying to access the variable.
help please
Thanks for this post. It quickly helped me figure out an issue that I had with accessing a C# script from js. This is interesting, too, b/c I just learned yesterday that you can do without the quotes when you use GetComponent this way:
csScript = this.GetComponent(“CSharp1”);
b/c this also works: csScript = this.GetComponent(CSharp1);
… in this case, you’re getting the component by type. Just saves a little typing.
Thanks! Good to know that now, it’s possible to obtain a C# script Component by type in JavaScript. It wasn’t possible to do that in Unity 2.6.
I have done everything needed to access a c# script using java but it continually gives me this error. any thoughts?
“The name ‘FollowAI’ does not denote a valid type (‘not found’).”
mee too :(
Thanks.
You’re so awesome!
Hi. Very nice tutorial.
I have a simple javascript file with a static variable. This javascript file is not attached to any gameobject. How do I access this static variable from other C# scripts ( w/ or w/o attaching to gameobjects)
Thanks very much for this tutorial, i had a similar problem. I had an object with a js attached, wich i tryed to access in a c# script (a boolean variable). Finaly it worked with this solution (maybe it helps someone else):
public class GameCtrl : MonoBehaviour {
…
private GameObject lvl_count;
private level_counter jsScript; // level_counter is a javascript located in Stadard Assets
…
void Start() { // can be an other function
…
lvl_count = GameObject.Find(“level_count”);
// “level_count” is a GameObject, which will be spawned in an other scene, with DontDestroyOnLoad command in the Awake() function
jsScript = lvl_count.GetComponent();
…
void Update(){
…
jsScript.doSomething();
…
}
its a behavior script … you suold delete the mono behavior and create it like a class
You are my King tank you So much !!!!
really apricate you for that Code, keep it on :]
Omer.
It’s nearly impossible to find educated people for this topic, but
you sound like you know what you’re talking
about! Thanks