Extra life after a certain amount of points
Posted by Dimitri | Oct 25th, 2010 | Filed under Featured, Programming
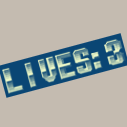
Finally, a post directly related to game programming! I will explain how to write a piece of code responsible for giving an extra life to the player’s character after a certain amount of points. The following code was written in Java, because it’s it would be shorter and easier to explain. Since this a generic algorithm, let’s assume that the game logic runs inside a method called Update() that is a member of the GameLogic class, responsible for our hypothetical game logic. Additionally, we need to have in mind that this method is being constantly called inside the game loop.
So here’s the code:
- //a bunch of imports
- public class GameLogic()
- {
- //the game score
- private int score;
- //number of lives
- private int numberOfLives;
- /*variables used to add a life after a certain score*/
- //add a life after 500 every points
- private int interval=500;
- //the extra life counter
- private int elCounter=1;
- //the game logic
- Update()
- {
- /*specific game code block beginning...*/
- //Game code goes here.
- /*specific game code end...*/
- //extra life check
- if(score >= interval * elCounter)
- {
- //add one to numberOfLives
- ++numberOfLives;
- //add one to the extra life counter
- ++elCounter;
- }
- }
- }
The logic behind this code is based on variables working as counters (elCounter and numberOfLives). Every time score value is greater than interval multiplied by elCounter a new life is added to numberOfLives and elCounter is incremented. By doing that, the new limit that the score is compared to inside the if statement gets raised.
In this example the code inside the if statement will only increment numberOfLives and elCounter after score value is greater or equal to 500. Then, it will only execute again if score is greater than 1000, because interval * elCounter => 500 * 2 = 1000. Then, one life will be added after 1500, 2500, 3000 points and so on…
This code can be easily extended to create a combo system. Just set interval to a lower and more appropriate initial value and create the game’s specific if statements to check whether the score should be incremented or reset back to 0.
Thank you so much. I am using javascript in unity but this has been the most useful script idea for this that I could find!!
Hey I think and easier way is to use the mod(%) operator. (if score % 500 = 0) will always return 0 if it’s an increment of 500. so 500, 1000, 1500 etc will always be 0 and everything else will be a value.