Posts Tagged ‘Code Snippet’
Posts that have small parts from a bigger code.
Unity3D: JavaScript->C# or C#->JavaScript access
Posted by Dimitri | Filed under Featured, Programming
When programming for Unity3D, there are some cases where we need to access a script written in another programming language that isn’t the one we are currently using. Although it is highly recommended to convert all scripts to the same one, it is useful to know how to access a C# script from a JavaScript class and the other way around.
The first thing to do is place the scripts in the correct folders in your Project tab. The script you want to have access must be inside the Standard Assets or the Plugins folder. The other script has to be placed outside these folders. After this step, just call the GetComponent() method as any other Component. Here’s a JavaScript example: (more…)
Android: Changing the ‘back’ button behaviour
Posted by Dimitri | Filed under Programming
Sometimes, when programming Android applications, there is the need to assign another behavior for the ‘Back’ button that isn’t the default one. Although not recommended, there are some cases that changing the ‘Back’ button behavior is necessary, such as to avoid accidentally finishing the current Activity.
For example, a text editor, should confirm if the user really wants to quit without saving the current changes, or a game, that check if it is the player’s intention to forfeit the current game session.
So, to start changing the ‘Back’ button, behavior, you need to override the onKeyDown() method and than check if the desired button has been pressed: (more…)
Android OpenGL: Get the ModelView Matrix on Cupcake (1.5)
Posted by Dimitri | Filed under Programming
One of the most annoying things when developing apps with OpenGL in Android 1.5, is the fact that there’s no access to the ModelView or the Projection matrices. It is not possible to call the glGetFloatv function because it wasn’t implemented until OpenGL ES 1.1, which isn’t available in the Cupcake versions. So, how to get the ModelView matrix on Android 1.5? The first thing you are going to do is grab these three classes: MatrixGrabber, MatrixStack and MatrixTrackingGL. All of them are inside the API demos, under the package com.example.android.apis.graphics.spritetext.
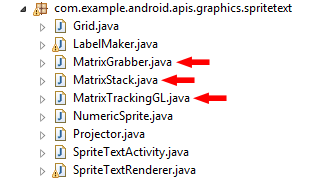
You're going to need these.
Unity3D: JavaScript vs. C# – Part 1
Posted by Dimitri | Filed under Featured, Programming
This is the first post of a series that will discuss some differences between JavaScript and C# when programming for Unity3D game engine. A project with all the scripts used in these posts will be available for download in the last post of the series. So let’s start from the start and see some of the main differences between the two languages.
The first and most easily distinguishable is the notation used to declare variables and methods. When programming in JavaScript, it isn’t necessary to declare the variable or method type whether in C#, it’s a must. That is because JavaScript is a weakly typed language, meaning that the interpreter chooses the best type to use at compilation-time. C# is a totally different because it is strongly typed, so, the type has to be declared before a variable or method. Let’s see some examples to better grasp this concept. The following script is a JavaScript example: (more…)
Android OpenGL: Texture from Canvas
Posted by Dimitri | Filed under Programming
Another post about Android programming, although this time, it’s going to incorporate some OpenGL techniques. The code below shows how to draw a Canvas into a Bitmap, and then, load it as a OpenGL texture object. This means that it is possible to use all Canvas methods to draw into a texture, like drawCircle(), drawPoints() or drawText(). This is useful to render text to a texture and to dynamically generate textures.
So here’s the code: (more…)