Posts Tagged ‘Android Programming’
Android: Changing the ‘back’ button behaviour
Posted by Dimitri | Filed under Programming
Sometimes, when programming Android applications, there is the need to assign another behavior for the ‘Back’ button that isn’t the default one. Although not recommended, there are some cases that changing the ‘Back’ button behavior is necessary, such as to avoid accidentally finishing the current Activity.
For example, a text editor, should confirm if the user really wants to quit without saving the current changes, or a game, that check if it is the player’s intention to forfeit the current game session.
So, to start changing the ‘Back’ button, behavior, you need to override the onKeyDown() method and than check if the desired button has been pressed: (more…)
Android OpenGL: Get the ModelView Matrix on Cupcake (1.5)
Posted by Dimitri | Filed under Programming
One of the most annoying things when developing apps with OpenGL in Android 1.5, is the fact that there’s no access to the ModelView or the Projection matrices. It is not possible to call the glGetFloatv function because it wasn’t implemented until OpenGL ES 1.1, which isn’t available in the Cupcake versions. So, how to get the ModelView matrix on Android 1.5? The first thing you are going to do is grab these three classes: MatrixGrabber, MatrixStack and MatrixTrackingGL. All of them are inside the API demos, under the package com.example.android.apis.graphics.spritetext.
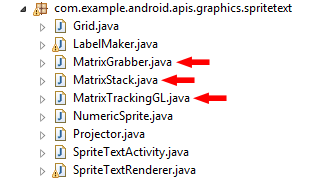
You're going to need these.
Android OpenGL: Texture from Canvas
Posted by Dimitri | Filed under Programming
Another post about Android programming, although this time, it’s going to incorporate some OpenGL techniques. The code below shows how to draw a Canvas into a Bitmap, and then, load it as a OpenGL texture object. This means that it is possible to use all Canvas methods to draw into a texture, like drawCircle(), drawPoints() or drawText(). This is useful to render text to a texture and to dynamically generate textures.
So here’s the code: (more…)
How to get Android local files URI
Posted by Dimitri | Filed under Programming
When programming applications for Android that requires the playback of audio or video files, sometimes, there’s the need to obtain the URI of those media files instead of using a String for the absolute path. But what is a URI? A URI (Uniform Resource Identifier) is an address to an local or internet resource. It’s more like a standardized path syntax that allows pointing to a specific resource that’s available over the internet, however we are going to use it to point it to a local resource.
A URI is specially useful, when using the VideoView class to load a video located on the res folder or in the SD card. Passing the video file to the VideoView as a String won’t even work on an emulated Android device. This way, we need to get the URI of the file.
Access Activity class from View
Posted by Dimitri | Filed under Featured, Programming
So, it begins! This is the first on this blog!
Let’s start with a Android Programming tip: how to access the Activity from an instantiated View object.
The first thing to do is consider if you really need to call an Activity from a View. Take some time to analyze your classes and their relationships. Probably, the variable/method you need to access doesn’t have to be a member of the Activity class. In that case, think about placing that variable/method inside the View or create a new class that has variables/methods which can be accessed by both Activity and View. Unless an Activity variable or method (e.g. Activity.finish() ) needs to be called from the View, there is no need to try to access the Activity from the current View.
But if you need to access a method/variable of the calling Activity from the View, here is how it’s done: