Unity: assigning a texture to the cursor
Posted by Dimitri | Nov 19th, 2012 | Filed under Programming
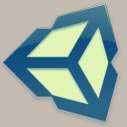
Unity 4.0 has been released last week and there are tons of new useful features to explore. One of those new fresh features is the option to set a Texture2D as a cursor. Although the code featured at the official documentation is extremely simple and explains how to achieve that with the OnMouseEnter() and the OnMouseExit() method, it will only work when the cursor is colliding with the 3D object in screen space. However, what if the game requires a default customized cursor that lasts throughout the whole level (or game)?
To set a Texture2D as the cursor throughout the duration of a level, the only thing that needs to be done is call the static Cursor.SetCursor method and pass the texture as the first parameter. Therefore, following the MonoBehaviour class logic, if the Cusor.SetCursor() method is called at the Start() or Awake() methods, this should replace the current cursor with the one specified by the Texture2D passed as the first parameter. Although this is the most straightforward approach, it doesn’t work by the time this tutorial is being written.
The Texture2D will be set as the cursor just for a couple of frames, and then, reset to the system’s current cursor. To avoid that, simply use the following script that delays the SetCursor() method call by placing it inside another method and delaying it’s call at initialization :
using UnityEngine; using System.Collections; public class CustomCursor : MonoBehaviour { //The texture that's going to replace the current cursor public Texture2D cursorTexture; //This variable flags whether the custom cursor is active or not public bool ccEnabled = false; void Start() { //Call the 'SetCustomCursor' (see below) with a delay of 2 seconds. Invoke("SetCustomCursor",2.0f); } void OnDisable() { //Resets the cursor to the default Cursor.SetCursor(null, Vector2.zero, CursorMode.Auto); //Set the _ccEnabled variable to false this.ccEnabled = false; } private void SetCustomCursor() { //Replace the 'cursorTexture' with the cursor Cursor.SetCursor(this.cursorTexture, Vector2.zero, CursorMode.Auto); Debug.Log("Custom cursor has been set."); //Set the ccEnabled variable to true this.ccEnabled = true; } }
The above script features two public variables: one Texture2D, for the cursor and a boolean, that tells whether the custom cursor is set or not (lines 7 and 10). This boolean isn’t a requirement to make this script work, however, it’s a good idea to have a variable that flags whether the current cursor has changed or not. That way, if necessary, it’s possible to verify what is the current cursor texture from outside the script.
Skipping to the bottom of the script, the SetCustomCursor() method is being defined (lines 26 through 33). Inside it, the Cursor.SetCursor() method is called. It takes three parameters (line 29). The first one is a Texture2D that’s going to replace the current cursor. The second, a Vector2, is the offset of the target point – an adjustment to the position registered by a click. Finally, the third parameter tells that Unity should detect if the cursor that we want to replace is a hardware or software cursor.
Other than that, this method prints a message to the console to confirm that the current cursor has been defined as the Texture2D and the sets the ccEnabled to true (lines 30 and 32). Back to the top of this script, the Start() method just invokes the SetCustomCursor() method with a delay of two seconds (line 15).
The OnDisable() method just resets the current cursor to the system default by passing null as the Texture2D to the Cursor.SetCursor() method (lines 18 through 24).
That’s it! Don’t forget to assign the Texture2D at the Inspector:
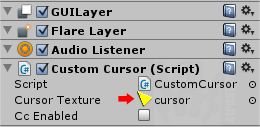
Drag and drop a texture to be used as the cursor.
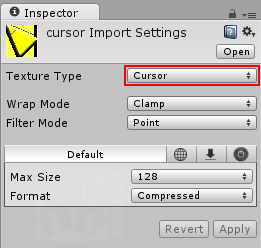
Don’t forget to select Cursor as the Texture Type at the Texture Importer.
Final Thoughts
By using the above script, the cursor changes will persist throughout the whole level, if the script remains enabled. To make it the default cursor texture during a whole game session, just attach this script to an empty game object and add a call to the DontDestroyOnLoad() at the beginning of the script.
Also, if the value of ccEnabled is important to the game’s logic, make it private and add public properties for obtaining it’s current value. I haven’t done this in the above script for the sake of simplicity. It would only be an additional concept to explain that doesn’t affect the script’s logic.
The above script has been tested on a Windows 7 machine. Regardless of the original texture size, when assigned to a cursor, it’s being rendered with a size of 32×32 pixels. I have no idea why this happens. Also, by the time this text was written, Unity’s cursor support is currently available only for Windows and Mac machines. I don’t know what size the cursor will be rendered on Macs or other Windows variants.
If you find out the custom texture cursor size on Mac computers or have anything else to say that might improve this post, please leave a comment below.
Downloads
Here’s the sample project. The script has been attached to the ‘Main Camera’. A JavaScript version has also been included:
Be the first to leave a comment!