Android: Loading files from the Assets and Raw folders
Posted by Dimitri | May 24th, 2011 | Filed under Programming
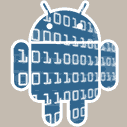
This tutorial will explain how to load files from the res/raw and the Assets folder using a String to specify the file name. Yes, there are a lot of tutorials on this subject, but they all use the automatically generated integer IDs from the R class as inputs and not many of them even mention the possibility of loading files from the Assets folder. As a result of depending on the ID, the file reference must be known beforehand.
Instead, the code featured in this post will explain how to find the reference to the file and then load it at runtime based solely on its name. This means that the reference ID and the file don’t even have to exist in the first place, and can be acquired at run time.
Before looking into the code, it’s important to highlight the main differences between the raw folder and the Assets folder. Since raw is a subfolder of Resources (res), Android will automatically generate an ID for any file located inside it. This ID is then stored an the R class that will act as a reference to a file, meaning it can be easily accessed from other Android classes and methods and even in Android XML files. Using the automatically generated ID is the fastest way to have access to a file in Android.
The Assets folder is an “appendix” directory. The R class does not generate IDs for the files placed there, so its less compatible with some Android classes and methods. Also, it’s much slower to access a file inside it, since you will need to get a handle to it based on a String. There is also a 1MB size limit for files placed inside the Assets folder, however some operations are more easily done by placing files in this folder, like copying a database file to the system’s memory. There’s no (easy) way to create an Android XML reference to files inside the Assets folder.
Here’s the code:
package fortyonepost.com.lfas;//Created by DimasTheDriver Apr/2011. import java.io.ByteArrayOutputStream; import java.io.IOException; import java.io.InputStream; import android.app.Activity; import android.content.res.Resources; import android.os.Bundle; import android.util.Log; import android.widget.Toast; public class LoadFromAltLoc extends Activity { //a handle to the application's resources private Resources resources; //a string to output the contents of the files to LogCat private String output; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); //get the application's resources resources = getResources(); try { //Load the file from the raw folder - don't forget to OMIT the extension output = LoadFile("from_raw_folder", true); //output to LogCat Log.i("test", output); } catch (IOException e) { //display an error toast message Toast toast = Toast.makeText(this, "File: not found!", Toast.LENGTH_LONG); toast.show(); } try { //Load the file from assets folder - don't forget to INCLUDE the extension output = LoadFile("from_assets_folder.txt", false); //output to LogCat Log.i("test", output); } catch (IOException e) { //display an error toast message Toast toast = Toast.makeText(this, "File: not found!", Toast.LENGTH_LONG); toast.show(); } } //load file from apps res/raw folder or Assets folder public String LoadFile(String fileName, boolean loadFromRawFolder) throws IOException { //Create a InputStream to read the file into InputStream iS; if (loadFromRawFolder) { //get the resource id from the file name int rID = resources.getIdentifier("fortyonepost.com.lfas:raw/"+fileName, null, null); //get the file as a stream iS = resources.openRawResource(rID); } else { //get the file as a stream iS = resources.getAssets().open(fileName); } //create a buffer that has the same size as the InputStream byte[] buffer = new byte[iS.available()]; //read the text file as a stream, into the buffer iS.read(buffer); //create a output stream to write the buffer into ByteArrayOutputStream oS = new ByteArrayOutputStream(); //write this buffer to the output stream oS.write(buffer); //Close the Input and Output streams oS.close(); iS.close(); //return the output stream as a String return oS.toString(); } }
The first thing the code does is to create two private variables. A Resources object that will act as a handle to the app’s resources and a String, that will be used to output the content of the files to LogCat (lines 16 and 18).
Now let’s jump straight to line 60 where the LoadFile() method is defined. It returns a String and takes two parameters: the first one is the file name and the second is a boolean, that signals the method whether it should load from the res/raw or the Assets folder.
After that, the method creates a InputStream object (line 63). Streams are like handles to read files into buffers (Input Stream) and to write files from these buffers (Output stream).
Line 65 checks if the loadFromRawFolder parameter is true. Case it is, the code at lines 68 and 70 is executed. The former dynamically loads resources from the raw folder. The getIdentifier() method from the resources object returns a resource ID based on the path. This parameter is composed by:
package name:type of resource/file name
Package name is self explanatory; type of resource can be one of the following: raw, drawable, string. File name, in this example, is the fileName parameter, an it’s being concatenated to create one single String. Since all information that this method requires is being passed on the first parameter the other two can be null.
Finally, line 70 feeds this ID to the openRawResource() method, which will return a InputStream from a file located at the res/raw folder.
At the else block, the Assets folder is being opened, by first calling the getAssets() method to return a handle to the Assets folder and right after that, the open() method is called, passing the fileName as the parameter. All that is done to also return the InputStream, although this time, for a file at the Assets folder (line 75).
Now that we have the InputStream, we can create the buffer to read the file into. That’s accomplished by line 79, that creates a byte array that has exactly the same length as iS (the InputStream object). The file is read into the buffer at the next line (line 81).
With the file loaded into the buffer, it’s time to prepare a OutputStream to write the buffer contents into. First, a object of this type is created at line 83. Then, the write() method is called passing the buffer as the parameter (line 85). With that, a handle to the file’s content was created.
There’s nothing left to do with these two streams, so they are closed at lines 87 and 88. Finally, the return for this method is set, by converting the oS object to a String (line 91).
At last, the LoadFile() method is called at line 33 (don’t forget to omit the file extension) and at line 47 (don’t forget to include the file extension). Both method calls are surrounded by a try/catch block since they can throw an exception.
The returned String from the method calls are stored at the output variable. It’s then later used to print the contents of the loaded files into LogCat’s console (lines 35 and 49).
And that’s about it! The method that was declared in the Activity can be easily adapted to load and return anything from these folders, not just a String. Additionally, it can be used to dynamically to acquire a reference, and load files at run time.
even though that this post is kinda old .. I’ve been looking for a way to do this
thanx a lot XD
Hello DtheD,
Thank you for a very educational tutorial. I am new in this Android venture and trying to learn as much as I can.
Thank you so much! This kind of tutorial (or anything related to using Assets) is very rare on the net, even on the Android Dev Guide. It’s exactly what I was looking for :)
Hey is it possible to copy a database that is about 20mb to the android file system. If not pls tell me a recomended way to copy larger db to the android file system.
I think this link might be helpful:
Load files bigger than 1M from assets folder
iS.available() is *not* guaranteed to give you the actual size of the bytes being read. The docs warn you specifically not to allocate a buffer based on the return value from this function.
Perfect… thnx….
If i want code to load Large images from server (any site)
which size can be 1mb or more than 1mb
I have tried but it crashes my application
can any one help me ?
if you have code for that then please mail me
on gtumca@yahoo.com
Nice blog post, it’s very useful for me and also my android developer friends…
Can u please say me how to get all the names of the file and their ID’s from raw file. Because I want to develop to image Viewer where right now I am able to show the images statically. But I want to show them dynamically
Very nice blog. Thankx for sharing your knowledge. Also, i have one question? is that possible to add some images into Assets at Run time and load that images?
hi i need to load hashed file containing images, sounds, textures this file is already compressed to a 66MB file size. When i read header information from the file i get pieces of gibberish (header information is not compressed). The header information contains file locations to data within the large file.
Any Ideas why?
I think that happened because there’s a size limit to files placed inside the Assets folder. By the time this post had been written, the limit was 1MB.
Hello, i feel that i noticed you visited my website thus
i came to return the prefer?.I am attempting
to to find issues to enhance my website!I suppose its adequate to make use of a few of your ideas!!