Android OpenGL: Get the ModelView Matrix on Cupcake (1.5)
Posted by Dimitri | Nov 25th, 2010 | Filed under Programming
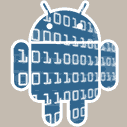
One of the most annoying things when developing apps with OpenGL in Android 1.5, is the fact that there’s no access to the ModelView or the Projection matrices. It is not possible to call the glGetFloatv function because it wasn’t implemented until OpenGL ES 1.1, which isn’t available in the Cupcake versions. So, how to get the ModelView matrix on Android 1.5? The first thing you are going to do is grab these three classes: MatrixGrabber, MatrixStack and MatrixTrackingGL. All of them are inside the API demos, under the package com.example.android.apis.graphics.spritetext.
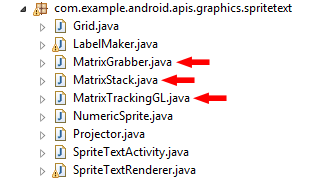
You're going to need these.
Get these classes and add them to the package that contains the Activity which sets your GLSurfaceView object as the current View. A lot of errors will occur, mostly because of naming issues and imports. Just fix these errors and let’s start programming.
Inside that Activity, you will have to call the setGLWrapper() method of your GLSurfaceView object. This method takes a GLWrapper interface object as a parameter. Setting this wrapper makes it possible to get values from the ModelView matrix of the current OpenGL interface object. Here is the code:
//creates a OpenGL surface view private GLSurfaceView glSV; /** Called when the activity is first created. **/ @Override public void onCreate(Bundle savedInstanceState) { //sets the Bundle super.onCreate(savedInstanceState); //Instantiates the GLSurfaceView glSV = new GLSurfaceView(this); //sets the wrapper glSV.setGLWrapper(new glSV.GLWrapper() { public GL wrap(GL gl) { return new MatrixTrackingGL(gl); } }); }
With these lines of code, we have set the GLWrapper that will return a MatrixTrackingGL object. After doing that, we can get the ModelView or the Projection matrices, by instantiating a MatrixGrabber object, and them, passing the OpenGL interface as a parameter to its methods. You can do it inside your GLSurfaceView class. Here’s an example:
//create the matrix grabber object in your initialization code private MatrixGrabber mg = new MatrixGrabber(); //and them, call the getCurrentModelView() for the ModelView matrix: mg.getCurrentModelView(gl); //values are stored at the mg.mModelView array, so: Log.i("Translation Z value", Float.toString(mg.mModelView[14]));//prints the current translation z value //to get the current Projection matrix: mg.getCurrentProjection(gl); //values are stored at the mProjection, so: Log.i("First projection matrix value", Float.toString(mg.mProjection[0]));//prints the first projection matrix value
And that’s all there is to it.
hi,
simple and efficient explanations.
after some trouble, I got it to work flawlessly :)
thanks a bunch
Thanks for the article..
helped alot.!
great !!!
thanks a lot !